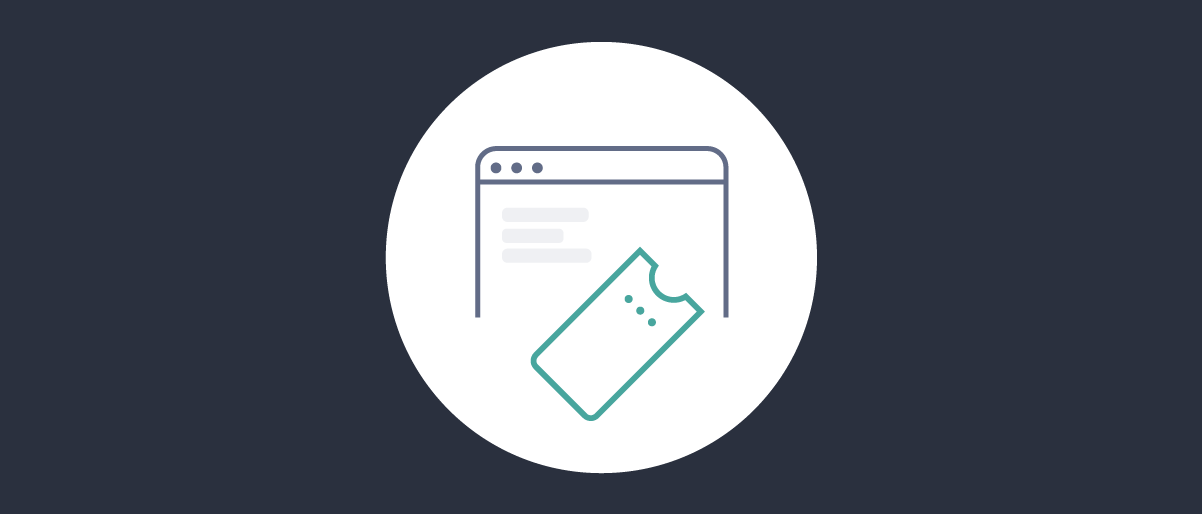
Token Handler Development Setup
On this page
The token handler pattern follows security best practices for Single Page Applications (SPA). OpenID Connect is combined with an application cookie layer, and only the most secure HTTP-only SameSite=strict Secure
cookies are used in the browser. This is done without compromising the web architecture, or needing to write any cookie-related code.
The separation of concerns used in the token handler pattern does however add complexity to the development setup. In older website architectures, developers ran a web backend that managed static content, the OAuth client, and API routes together:
In the token handler pattern, the same concerns remain, but deployment is more separated. When adopting the token handler pattern, you need to start with some deployment work. This article describes some cloud native patterns you can use. Once complete, you will be able to write code in a pure SPA manner, while also running production level security on your workstation.
Docker Token Handler
The main components used are introduced in the token handler design overview. On a development computer, the simplest way to get started running them is to use Docker. A local API gateway then sits in front of the OAuth agent and manages forwarding JWT access tokens to APIs.
We provide a detailed deployment example that you can study to understand how each component is deployed and the main configuration settings. We explain how to run our example SPA in development mode, with web static content served by the webpack development server, with all API components deployed to Docker.
Development URLs
There are a few ways in which you can design URLs for development.
Localhost
The following deployment uses localhost URLs, with port 3000 for a local web static content host and port 3001 for the API gateway behind which the token handler components are hosted.
Same Origin
Although you could use localhost
URLs, we instead recommended using a more meaningful web origin for development. The simplest option is to invent a web domain name for local development, then add it to your computer's hosts file:
127.0.0.1 www.example-local.com
You can then use a local same origin deployment similar to the following production deployment, where all three web concerns are hosted together behind a gateway. The only difference during web development is that static content is served locally:
Same Site
Alternatively, you can add two local domains, to separate the web and API concerns more completely. The token handler components then run in the same parent domain as the SPA's web origin, using a prefix of your choice, such as bff
or api
.
127.0.0.1 www.example-local.com api.example-local.com
This development setup mirrors the following production deployment, where web static content is downloaded from a content delivery network. Of course, during web development, the static content will continue to be served locally:
Upgrade to a Remote Token Handler
Once deployment is understood, you can then use a DNS solution to avoid needing to run token handler components locally. To do so, run the same Docker deployment to push them to a shared environment, dedicated to enabling productive web development.
One option is to spin up a domain in your cloud platform, while adding an entry for the web domain to your hosts file. Either HTTP or HTTPS URLs could be used for a development setup. You then have a pure SPA web development setup, and you also operate with the same security as your customer users:
Cross Origin Requests
All of the development setups shown above serve static content from one process, while managing OAuth and API requests from other processes. This requires different ports or host names to be used during development. Requests from the SPA to the API gateway and OAuth agent are therefore cross origin. When calling APIs, the SPA will use CORS request and response headers. The Curity components manage this in the correct way.
Full Stack Setups
A Docker based deployment can remain useful, to provide finer control over custom individual setups, without impacting the rest of the web development team. For example, a developer might want to run both the local SPA and one or more APIs locally. To do so, that developer can simply route from the Docker API gateway back to the local computer, using the well known host name of host.docker.internal
:
Conclusion
The token handler pattern enables you to implement a secure cookie layer at the application level, while also following a pure SPA development model. This is done by separating web and API concerns, which in turn adds more moving parts to the development setup. Some initial investment in deployment is required, after which you can continue to focus only on web customer experiences, with the difficult security externalized.
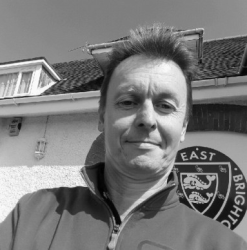
Gary Archer
Product Marketing Engineer at Curity
Join our Newsletter
Get the latest on identity management, API Security and authentication straight to your inbox.
Start Free Trial
Try the Curity Identity Server for Free. Get up and running in 10 minutes.
Start Free Trial