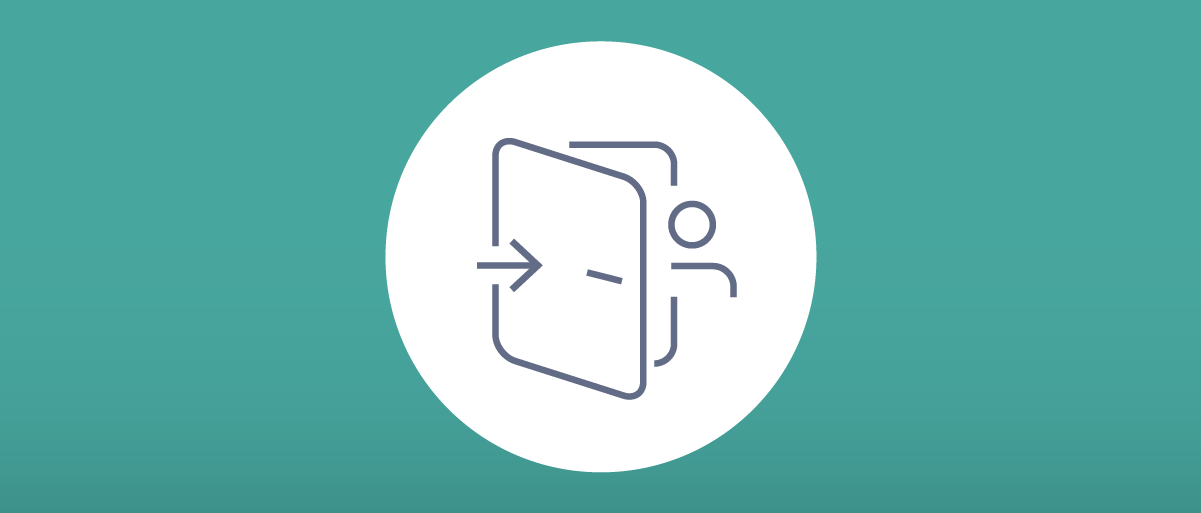
Client Initiated Backchannel Authentication (CIBA) Flow
On this page
Overview of the CIBA Flow
The Client Initiated Backchannel Authentication (CIBA) defines a protocol to support initiating authentication without user interaction from a Consumer Device. Authentication is performed via an Authentication Device by the user who also consents (if required) to the request. CIBA is also referred to as a decoupled flow. You can learn more about these concepts in the article Client Initiated Backchannel Authentication .
This article describes the messages in the Poll mode as specified by CIBA and aims to help developers understand and implement the specification.
- The flow starts with an Authentication Request to the Backchannel Authentication Endpoint. The Authorization Server immediately returns an Authentication Request Acknowledgement with a transaction identifier, the
auth_req_id
. - The user authenticates through an out-of-band mechanism, a mobile device in this example.
- Meanwhile, the client polls the Authorization Server regularly, checking for a token. The Authorization Server returns a Token Response with either an error indicating that the authentication is pending, or the tokens if available.
Metadata
At registration, the client specifies that it will use the Poll mode for token delivery. The client must support the grant type urn:openid:params:grant-type:ciba
.
Parameter | Mandatory | Description |
---|---|---|
grant_types | yes | A list of grant types to be used against the token endpoint. Must include urn:openid:params:grant-type:ciba for CIBA. |
token_delivery_mode | yes | The mode to use for token delivery. In this case, it'spoll . |
backchannel_user_code_parameter | no | Specifies if the client supports the user_code parameter. The default value is false. Only relevant if the Authorization Server supports user codes. |
token_endpoint_auth_method | yes | Public clients are not supported by CIBA. The client must authenticate with any of the client authentication methods supported by the Authorization Server. |
If utilizing Pairwise Pseudonymous Identifiers (PPID), the following parameters are of importance.
Parameter | Mandatory | Description |
---|---|---|
subject_type | yes | pairwise indicates that PPIDs are used in tokens |
jwks_uri | yes* | A URL to the client's public keys in the format of JSON Web Key Set. Either jwks_uri or sector_identifier_uri must be specified. |
sector_identifier_uri | yes* | A URL pointing to a file with a single JSON array of redirect_uri or jwks_uri values. If specified, the jwks_uri must be included in the list of URIs. |
Authentication Request
To initiate the Backchannel Authentication, the client sends an authentication request to the Authorization Server's Backchannel Authentication Endpoint using HTTP POST with Content-Type header set to application/x-www-form-urlencoded
. The client needs to authenticate to the Authorization Server as part of the authentication request. Any client authentication method defined by OpenID or extensions may be used.
Parameter Name | Mandatory | Description |
---|---|---|
scope | yes | CIBA extends OpenID Connect. The list of scopes in the authentication request must therefore contain openid . |
login_hint_token id_token_hint or login_hint | yes | A proprietary token, a previously issued ID token, or some other string that allows the Authorization Server to identify the user (who it requests to login). One of these parameters must be provided. |
acr_values | no | A space-separated list of preferred authentication context class references. The resulting ID token will contain an acr claim with the Authentication Context Class that the authentication satisfied. The acr claim value can specify the level of assurance of the user's identity or authentication method. |
binding_message | no | A human-readable message to be displayed at the consumption device and authentication device. This allows the user to ensure the transaction initiated by the consumption device is the same that triggers the action on the authentication device. |
user_code | no | If supported by the client and Authorization Server, the client may request a secret code from the user. This code will be used to authorize initiating an authentication at the user's authentication device. |
requested_expiry | no | Authentication requests have a lifetime, meaning they can time out if the user does not authenticate in a timely fashion. The client can influence the lifetime of the authentication request with this parameter. |
In the example below, the client uses mutual TLS, a client authentication method recommended by CIBA. When using mutual TLS for client authentication, the client must include its client_id
in the requests. The line breaks were added for display purposes.
POST /oauth/v2/oauth-authorize HTTP/1.1Host: idsvr.example.comContent-Type: application/x-www-form-urlencodedclient_id=consumer_device&scope=openid%20email%20phone&binding_message=2X56&requested_expiry=90&acr_values=urn%3Ase%3Acurity%3Aauthentication%3Abankid&login_hint_token=eyJhbGciOiJFUzI1NiJ9.eyJzdWJfaWQiOnsiZm9ybWF0IjoiZW1haWwiLCJlbWFpbCI6ImphbmVkb2VAZXhhbXBsZS5jb20ifX0.t5DsqWOhDH-Spbcx1TCytXjMq19C1QGgIXVk_ih-v3072yq673mpEBYhsISADwzrIe_0k-Si-PAcwJlONxhb2Q
Authentication Response
The Authorization Server authenticates the client and verifies the authentication request. It checks, for example, if the hint is valid and identifies a known user. In case of an error, an authentication error response is returned. Otherwise, the Authorization Server responds with a successful authentication request acknowledgment.
Successful Authentication Request Acknowledgement
The authentication request acknowledgment includes parameters that are of importance for upcoming requests.
Parameter Name | Mandatory | Description |
---|---|---|
auth_req_id | yes | This is the unique ID of the authentication request. It is used in the token requests to identify the transaction started by the client's authentication request. Thus, the client must keep this parameter. |
expires_in | yes | The number of seconds, since the authentication request was received, that the authentication request will be valid for. |
interval | no | The number of seconds that the client must wait between two polling requests. The default value is 5. |
Study the following non-normative example of an authentication request acknowledgment:
HTTP/1.1 200 OKContent-Type: application/jsonCache-Control: no-store{"auth_req_id": "bspuw6ea-scst-u5hn-p3nt-37khzwY4g","expires_in": 900,"interval": 5}
Authentication Error Response
If the authentication request raises an error, the Authorization Server will return an authentication error response using HTTP status codes and descriptive error codes in the body of the response. Just as with token error responses, the body is formatted as application/json
.
The following parameters appear in an error response:
Parameter Name | Mandatory | Description |
---|---|---|
error | yes | A predefined error code. |
error_description | no | Additional information about the error in a human-readable form to help developers diagnose the error. |
error_uri | no | A link to a human-readable web page with additional information about the error similar to error_description . |
The non-normative example below demonstrates an authentication error response as a result of the example authentication request listed above:
HTTP/1.1 400 Bad RequestContent-Type: application/json{"error": "unknown_user_id","error_description": "Cannot identify the subject from the login_hint_token."}
The Authorization Server may return one of the following errors. Please note that this list is not complete by any means. Refer to the specification for a complete list of error codes.
HTTP 400 Bad Request | |
---|---|
invalid_request | The request is malformed. This code may be returned if there is an error with the parameters, such as missing required parameters or duplicates. |
invalid_scope | The requested scope is invalid, unknown, or malformed. |
unknown_user_id | The Authorization Server cannot determine which user to authenticate based on the hint provided. |
invalid_binding_message | The binding message is not accepted by the Authorization Server because it is considered invalid in the context of the given authentication request. |
unauthorized_client | The client is not authorized to run a CIBA flow. |
HTTP 401 Unauthorized | |
---|---|
invalid_client | The client failed to authenticate. This may be, for example, due to invalid credentials or authentication methods. |
Token Request
In Poll mode, after having received the authentication request acknowledgment with auth_req_id
, the client polls the token endpoint at a reasonable interval. The client must not poll more frequently than the interval specified by the Authorization Server as part of the authentication acknowledgment.
The Authorization Server may use long polling and not respond before an authentication result is available or a timeout has occurred. Therefore, the CIBA specification recommends waiting for at least 30 seconds between two polling requests, which is the value recommended by RFC6202, Best Practices for Long Polling. When using long polling, the Authorization Server may take more time to respond than the specified interval. In this case, the client must not send two overlapping requests with the same auth_req_id
but wait for a response.
For the token request, the client forms a message using the application/x-www-form-urlencoded
format and sends it via HTTP POST to the token endpoint. The client must also authenticate as part of the request. The body of the token request contains at least the following parameters:
Parameter Name | Mandatory | Description |
---|---|---|
grant_type | yes | The flow to run to retrieve tokens. For CIBA, the value must be urn:openid:params:grant-type:ciba . |
auth_req_id | yes | The unique identifier for a transaction initiated by the client. The Authorization Server uses this identifier to retrieve the status of the authentication and issue tokens if authentication was successful and the user gave consent. |
The token request may look similar to this example. Note that the client uses mutual TLS for client authentication and must provide the client_id
.
POST /oauth/v2/oauth-token HTTP/1.1Host: idsvr.example.comContent-Type: application/x-www-form-urlencodedgrant_type=urn%3Aopenid%3Aparams%3Agrant-type%3Aciba&auth_req_id=bspuw6ea-scst-u5hn-p3nt-37khzwY4g&client_id=consumer_device
Successful Token Response
If the token request is valid, and if the user has been authenticated and has authorized the request, the Authorization Server will return a successful response just as with any other OpenID flow.
Consider the non-normative example below. Line breaks are added for readability.
HTTP/1.1 200 OKContent-Type: application/jsonCache-Control: no-cache, no-store{"access_token": "5ca69968-00ca-4535-82e3-c7badf90b51b","refresh_token": "0f8ed1e3-9542-45a0-84ce-4ae3ba61a60e","scope": "openid email phone","id_token": "eyJraWQiOiI2OTI0NjA1NjkiLCJ4NXQiOiJlYXM0bVVrOWpzMXRCZWVldzFtR3FkUW1RZHciLCJhbGciOiJSUzI1NiJ9.eyJleHAiOjE2MTkwNzk2MzIsIm5iZiI6MTYxOTA3NjAzMiwianRpIjoiYWQ2ZGZkNDYtZmZlMC00YmUyLTgzMDgtMGQ5NDgwODQ0NDkzIiwiaXNzIjoiaHR0cHM6Ly9pZHN2ci5leGFtcGxlLmNvbS9vYXV0aC92Mi9vYXV0aC1hbm9ueW1vdXMiLCJhdWQiOiJjb25zdW1lcl9kZXZpY2UiLCJzdWIiOiJqYW5lZG9lIiwiYXV0aF90aW1lIjoxNjE5MDc2MDMxLCJpYXQiOjE2MTkwNzYwMzIsInB1cnBvc2UiOiJpZCIsImF0X2hhc2giOiJ6QVNBWFQzdjVQUGh1TVRIdnF3QW9RIiwiZGVsZWdhdGlvbl9pZCI6ImFlNmIzZTIyLTcwMzAtNGJmYS05YWU2LWMxODIwYWI4NGE3MCIsImFjciI6InVybjpzZTpjdXJpdHk6YXV0aGVudGljYXRpb246YmFua2lkIiwic19oYXNoIjoiSTNtZ3VmV3JKb0JBdnJTNUJ4ZllKdyIsImF6cCI6ImNvbnN1bWVyX2RldmljZSIsImFtciI6InVybjpzZTpjdXJpdHk6YXV0aGVudGljYXRpb246YmFua2lkIiwicGhvbmVfbnVtYmVyIjoiKzEgKDMxMCkgMTIzLTQ1NjciLCJub25jZSI6IjE2MTkwNzUyNzkxODctNklwIiwiZW1haWwiOiJqYW5lZG9lQGV4YW1wbGUuY29tIiwic2lkIjoiSFJuVUtiY0szdjJ2MlcyZyJ9.U6gLsy7kPgIh5U9EFjdE-zprExfTwb5XY7sA95T0rvjfPWp7aF_Pzj7CNUAhhM50LSOVfVa0V0JHhfELuc5uh2D48vAVcRQtivAjMdq_Fmz5X46wZN87LpcrZsEENXWdR5Sh7CHorLcJrGoJStu4nMNRjUVS9KrQn5mKIj3s49y11BXhdAksxREEVDDvoI8TWSpT9_C1_6AuCWl-FGQZ6V5gAD8Q-qovqxmmbpn6UCOn0doJ05jC0JTOEd0fzdpchLSbRnhFxgp3aTgXwh80JKyNn5Itg4KUPnHjPCELz-xsoktpPcoasepgrkxHnswwGEG0RCBbRkhIPOkXt31G9Q","token_type": "bearer","expires_in": 300}
Token Error Response
As with any other flow in OpenID Connect, the token request in CIBA may result in an error. In addition to the error codes defined as part of the OAuth 2.0 specification, CIBA considers the following as applicable as well:
Error Code | Description |
---|---|
authorization_pending | The user has not yet been authenticated, and the authorization request is still pending. |
slow_down | The authorization request is still pending, and the client must increase the interval for polling requests by at least 5 seconds. |
expired_token | The transaction (auth_req_id ) has expired. The client must start over with a new Authentication Request. |
access_denied | The user did not consent to the authorization request. |
HTTP/1.1 400 Bad RequestContent-Type: application/jsonCache-Control: no-cache, no-store{"error": "authorization_pending"}
The specification extends the meaning of some error codes as follows:
Error Code | Description |
---|---|
invalid_grant | The provided auth_req_id is invalid or was issued to another client. |
invalid_request | The server may return this error if the client does not respect the polling interval. When receiving an invalid_request , the client must stop polling for the auth_req_id . |
Resources and Further Information
- OpenID Connect Client Initiated Backchannel Authentication Flow
- Error Response in OAuth 2.0
- Error Response in OAuth 2.0 Device Authorization Grant
- RFC6202 - Best Practices for Long Polling

Judith Kahrer
Product Marketing Engineer at Curity
Join our Newsletter
Get the latest on identity management, API Security and authentication straight to your inbox.
Start Free Trial
Try the Curity Identity Server for Free. Get up and running in 10 minutes.
Start Free Trial